C# PDFSharp生成pdf文件
2022-04-11 18:20
6718 人阅读

PdfSharp一款开源的用于创建,操作PDF文档的.Net类库,本文简单介绍使用方法
一. 添加NuGet程序包,搜索pdfsharp,安装
二. 主要操作类
1. PdfDocument : 表示一个PDF文档对象,调用save方法保存文档到指定路径。
2. PdfPage : 表示PDF文档中的一页。
3. XGraphics:表示页面上的绘制对象,所有的页面内容,都可通过此对象绘制。如:DrawString绘制文本内容,DrawLine绘制直线等。
4. XFont:绘制文本的字体,字体名称只能取C:\Windows\Fonts目录下的ttf字体文件,不能是ttc格式的字体。
5. XPen:绘制直线
6. XImage:绘制图片对象
7. XTextFormatter:表示一个简单的文本字体格式,如识别文本的换行符而实现自动换行等内容。
三. 制作pdf效果图
四. 核心代码
using PdfSharp.Drawing; using PdfSharp.Pdf; private static void PdfConvert() { try { // 创建新的PDF文档 PdfDocument document = new PdfDocument(); // 创建空页 PdfPage page = document.AddPage(); // 设置一个画布 XGraphics gfx = XGraphics.FromPdfPage(page); XFont font = new XFont("黑体", 20, XFontStyle.Bold); // 标题文本 gfx.DrawString("个人信息表", font, XBrushes.Black, new XRect(0, 50, page.Width, page.Height), XStringFormats.TopCenter); // 表格内框宽度 var tbW = (page.Width - 100) / 5; // 横线 使用画笔 + 位置信息 XPen pen1 = new XPen(XColor.FromKnownColor(XKnownColor.Black), 0.1); gfx.DrawLine(pen1, 50, 100, page.Width - 50, 100); XPen pen2 = new XPen(XColor.FromKnownColor(XKnownColor.Black), 0.1); gfx.DrawLine(pen2, 50, 130, page.Width - 50 - tbW, 130); XPen pen3 = new XPen(XColor.FromKnownColor(XKnownColor.Black), 0.1); gfx.DrawLine(pen2, 50, 160, page.Width - 50, 160); XPen pen4 = new XPen(XColor.FromKnownColor(XKnownColor.Black), 0.1); gfx.DrawLine(pen2, 50, 190, page.Width - 50, 190); // 竖线 XPen pens1 = new XPen(XColor.FromKnownColor(XKnownColor.Black), 0.1); gfx.DrawLine(pens1, 50, 100, 50, 190); XPen pens2 = new XPen(XColor.FromKnownColor(XKnownColor.Black), 0.1); gfx.DrawLine(pens2, 50 + tbW, 100, 50 + tbW, 190); XPen pens3 = new XPen(XColor.FromKnownColor(XKnownColor.Black), 0.1); gfx.DrawLine(pens3, 50 + tbW * 2, 130, 50 + tbW * 2, 160); XPen pens4 = new XPen(XColor.FromKnownColor(XKnownColor.Black), 0.1); gfx.DrawLine(pens3, 50 + tbW * 3, 130, 50 + tbW * 3, 160); XPen pens5 = new XPen(XColor.FromKnownColor(XKnownColor.Black), 0.1); gfx.DrawLine(pens5, 50 + tbW * 4, 100, 50 + tbW * 4, 160); XPen pens6 = new XPen(XColor.FromKnownColor(XKnownColor.Black), 0.1); gfx.DrawLine(pens6, 50 + tbW * 5, 100, 50 + tbW * 5, 190); // 文字 XFont font2 = new XFont("黑体", 16, XFontStyle.Regular); gfx.DrawString("姓名:", font2, XBrushes.Black, new XPoint(60, 120)); gfx.DrawString("电话:", font2, XBrushes.Black, new XPoint(60, 150)); gfx.DrawString("性别:", font2, XBrushes.Black, new XPoint(60 + tbW * 2, 150)); gfx.DrawString("联系地址:", font2, XBrushes.Black, new XPoint(60, 180)); XFont font3 = new XFont("黑体", 14, XFontStyle.Regular); gfx.DrawString("张飞", font3, XBrushes.Black, new XPoint(60 + tbW, 120)); gfx.DrawString("15800000000", font3, XBrushes.Black, new XPoint(60 + tbW, 150)); gfx.DrawString("男", font3, XBrushes.Black, new XPoint(60 + tbW * 3, 150)); gfx.DrawString("深圳市南山区粤海街道100号000楼", font3, XBrushes.Black, new XPoint(60 + tbW, 180)); // 图片 XImage image = XImage.FromFile(@"E:\本地\Demo\Demo\Demo.FrameWork\123456789.jpg"); gfx.DrawImage(image, 50 + tbW * 4 + 25, 105, 50, 50); // 保存文档 string filename = "个人信息.pdf"; document.Save(filename); } catch (Exception ex) { Console.WriteLine($"错误:{ex.Message}"); } }
和博主交个朋友吧
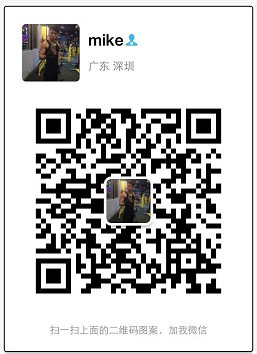