Asp.Net MVC 使用 Cropper进行图片裁剪
2018-01-17 22:57
2702 人阅读

项目中使用图片裁剪也是很常见的,一般上传文件的时候需要用户裁剪之后再保存文件。
这里介绍Jquery中Cropper在Asp.net MVC中进行裁剪
项目结构
首先需要引用文件
<link rel="stylesheet" href="/Content/cropper/dist/cropper.css" /> <link rel="stylesheet" href="/css/screencropper.css" /> <link href="/Content/bootstrap.min.css" rel="stylesheet" /> <script type="text/javascript" src="/scripts/jquery-1.10.2.min.js"></script> <script type="text/javascript" src="/scripts/bootstrap.min.js"></script> <script type="text/javascript" src="/Content/cropper/dist/cropper.js"></script> <script type="text/javascript" src="/Js/screencropper.js"></script>
这里 screencropper.css和screencropper.js是自定义文件
screencropper.css
.avatar-view { display: block; width: 220px; border: 3px solid #fff; border-radius: 5px; box-shadow: 0 0 5px rgba(0,0,0,.15); cursor: pointer; overflow: hidden; } .avatar-view img { width: 100%; } .avatar-wrapper { height: 364px; width: 625px; margin-top: 15px; box-shadow: inset 0 0 5px rgba(0,0,0,.25); background-color: #fcfcfc; overflow: hidden; } .avatar-wrapper img { display: block; height: auto; max-width: 100%; } .avatar-preview { float: left; margin-top: 15px; margin-right: 15px; border: 1px solid #eee; border-radius: 4px; background-color: #fff; overflow: hidden; } .preview-lg { height: 184px; width: 184px; margin-top: 15px; } .avatar-btns { margin-top: 30px; margin-bottom: 15px; } .avatar-btns .btn-group { margin-right: 5px; }
screencropper.js
$(function () { //'use strict'; function CropAvatar($element) { this.$container = $element; this.$avatarView = this.$container.find('.avatar-view'); this.$avatar = this.$avatarView.find('img'); this.$avatarModal = this.$container.find('#avatar-modal'); this.$avatarForm = this.$avatarModal.find('.avatar-form'); this.$avatarData = this.$avatarForm.find('.avatar-data'); this.$avatarSave = this.$avatarForm.find('.avatar-save'); this.$avatarWrapper = this.$avatarModal.find('.avatar-wrapper'); this.$avatarPreview = this.$avatarModal.find('.avatar-preview'); this.init(); } CropAvatar.prototype = { constructor: CropAvatar, init: function () { this.initModal(); this.addListener(); }, addListener: function () { this.$avatarView.on('click', $.proxy(this.click, this)); this.$avatarSave.on('click', $.proxy(this.completeCropper, this)); }, initModal: function () { this.$avatarModal.modal({ show: false }); }, initPreview: function () { var url = this.$avatar.attr('src'); this.$avatarPreview.empty().html('<img src="' + url + '">'); }, click: function () { this.$avatarModal.modal('show'); this.initPreview(); this.url = this.$avatar.attr('src'); this.startCropper(this.url); }, startCropper: function (url) { var _this = this; this.url = url; if (this.active) { this.$img.cropper('replace', this.url); } else { this.$img = $('<img src="' + this.url + '">'); this.$avatarWrapper.empty().html(this.$img); this.$img.cropper({ aspectRatio: 1, preview: this.$avatarPreview.selector, strict: false, crop: function (data) { var json = [ '{"x":' + data.x, '"y":' + data.y, '"height":' + data.height, '"width":' + data.width, '"rotate":' + data.rotate + '}' ].join(); _this.$avatarData.val(json); } }); this.active = true; } }, stopCropper: function () { if (this.active) { this.$img.cropper('destroy'); this.$img.remove(); this.active = false; } }, completeCropper: function () { var paramData = JSON.parse(this.$avatarData.val()); //进入后台进行图片裁剪 var paramCrip = { FileName: this.$avatar.attr('src'), X: Math.floor(paramData.x), Y: Math.floor(paramData.y), CropHeight: Math.floor(paramData.height), CropWidth: Math.floor(paramData.width), MaxHeight: Math.floor(paramData.height), MaxWidth: Math.floor(paramData.width) }; var that = this; $.ajax({ url: '/api/cropper/cropperimg', type: 'POST', dataType: 'json', data: paramCrip, success: function (data) { $("#ImgCropperPreview").attr("src", data.Url); that.cropDone(); } }); return false; }, cropDone: function () { this.$avatarForm.get(0).reset(); this.$avatar.attr('src', this.url); this.stopCropper(); this.$avatarModal.modal('hide'); } }; $(function () { return new CropAvatar($('#crop-avatar')); }); });
前端Html代码
<div class="container" id="crop-avatar"> <div> <div>裁剪后图片</div> <img id="ImgCropperPreview" src="/images/0f7f70a3bca44ab19305a73a4b07cd62.jpg" style="width:100px;height:100px;" alt="" /> </div> <div class="avatar-view" title="点击进行裁剪"> <img src="/images/0f7f70a3bca44ab19305a73a4b07cd62.jpg" alt="Avatar"> </div> <div class="modal fade" id="avatar-modal" aria-hidden="true" aria-labelledby="avatar-modal-label" role="dialog" tabindex="-1"> <div class="modal-dialog modal-lg"> <div class="modal-content"> <form class="avatar-form"> <div class="modal-header"> <button class="close" data-dismiss="modal" type="button">×</button> <h4 class="modal-title" id="avatar-modal-label">图片裁剪</h4> </div> <div class="modal-body"> <div class="avatar-body"> <div class="avatar-upload" style="display:none"> <input class="avatar-src" name="avatar_src" type="hidden"> <input class="avatar-data" name="avatar_data" type="hidden"> </div> <div class="row"> <div class="col-md-9"> <div class="avatar-wrapper"></div> </div> <div class="col-md-3"> <div class="avatar-preview preview-lg"></div> <div class="" style="text-align: center;margin-top: 5px;color: #f00;">当前尺寸:<span class="avatar-preview-size"></span></div> </div> </div> <div class="row avatar-btns"> <div class="col-md-3"> <button class="btn btn-primary btn-block avatar-save">提交</button> </div> </div> </div> </div> </form> </div> </div> </div> </div>
后端Api接口
public class CropperController : ApiController { #region 裁剪图片 /// <summary> /// 裁剪图片 /// </summary> /// <param name="dto"></param> /// <returns></returns> [HttpPost] public ResMsgModel CropperImg(FileScreenShotInfo dto) { ResMsgModel resMsgModel = new ResMsgModel(); dto.FileName = Path.GetFileName(dto.FileName); string newFileName = FileHelper.CropperImage(dto); resMsgModel.Success = false; resMsgModel.Url = "/Images/" + newFileName; return resMsgModel; } #endregion } public class ResMsgModel { public bool Success { set; get; } public string Url { set; get; } }
裁剪核心代码
public class FileHelper { /// <summary> /// 裁剪图片并保存 /// </summary> public static string CropperImage(FileScreenShotInfo dto) { string absolutePath = HostingEnvironment.MapPath("/Images"); string fileTempPath = Path.Combine(absolutePath, dto.FileName); Image originalImage = Image.FromFile(fileTempPath); string fileName = ""; Bitmap b = new Bitmap(dto.CropWidth, dto.CropHeight); try { using (Graphics g = Graphics.FromImage(b)) { //清空画布并以透明背景色填充 g.Clear(Color.Transparent); //在指定位置并且按指定大小绘制原图片的指定部分 g.DrawImage(originalImage, new Rectangle(0, 0, dto.CropWidth, dto.CropHeight), dto.X, dto.Y, dto.CropWidth, dto.CropHeight, GraphicsUnit.Pixel); Image displayImage = new Bitmap(b, dto.MaxWidth, dto.MaxHeight); string extensionName = Path.GetExtension(dto.FileName); fileName = Guid.NewGuid().ToString("N") + extensionName; string filePath = Path.Combine(absolutePath, fileName); displayImage.Save(filePath); } } catch (Exception e) { fileName = dto.FileName; } finally { originalImage.Dispose(); b.Dispose(); } return fileName; } } public class FileScreenShotInfo { /// <summary> /// 需要被裁剪的文件名(111.jpg) /// </summary> public string FileName { get; set; } /// <summary> /// 缩略图宽度 /// </summary> public int MaxWidth { get; set; } /// <summary> /// 缩略图高度 /// </summary> public int MaxHeight { get; set; } /// <summary> /// 裁剪宽度 /// </summary> public int CropWidth { get; set; } /// <summary> /// 裁剪高度 /// </summary> public int CropHeight { get; set; } /// <summary> /// X轴 /// </summary> public int X { get; set; } /// <summary> /// Y轴 /// </summary> public int Y { get; set; } }
效果截图
源码下载地址: http://download.csdn.net/download/jx_521/10211004
和博主交个朋友吧
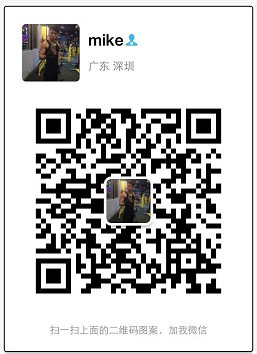